With the advent of more powerful processing, programming has split into two camps: synchronous and asynchronous. Each has its own use cases and pitfalls, and it’s important to know their differences so you can correctly choose the right type of communication in your projects.
Let’s demystify the whole concept of synchronous vs asynchronous programming.
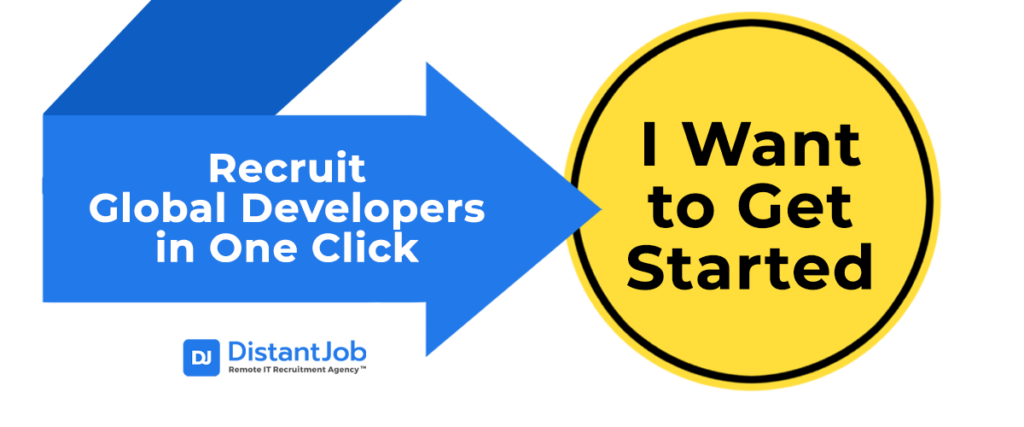
What Does Synchronous Mean In Programming?
Synchronicity in programming happens when the execution of operations is done sequentially. An operation will only be executed after the previous one is done.
This means that a potentially complex task may take a while before all its steps are executed and completed. In technical terms, synchronous calls are done in a single thread, and every operation being executed blocks that thread for the execution’s duration.
As a real-life metaphor, consider the preparation of a meal. When a person is alone preparing a meal, they will have to do all the tasks by themselves. And some steps require food processed in previous steps. You can rearrange the order slightly, but some steps have a fixed order. You can only mince a carrot after you’ve unpeeled it, for example.
What Does Asynchronous Mean?
While synchronous processing is done sequentially and in a specific order, asynchronous processing is done in parallel. Tasks that are not dependent on others can be offloaded and executed at the same time as the main operation and then report back the result when they are done.
Continuing our metaphor of preparing a meal, adding another cook to the kitchen allows for more time efficiency. While one person is taking care of chopping vegetables, another can start making the sauce. By adding yet another cook, they can prepare the meat while the other two are working. And so on. There are still some steps that need to happen in sequence (the assembly of the dish after every ingredient is prepared is an obvious one), but others can be carried out independently to speed up the process.
What Is The Difference Between Synchronous And Asynchronous?
The main difference when talking about operation synchronicity is in their thread usage. Synchronous processing uses only one thread where it executes all operations in succession. In contrast, each asynchronous operation is done in a different thread that reports back to the main thread with the result when complete or with an error in case of failure, leaving that thread open to process other requests.
When talking about synchronous vs asynchronous communication programming, synchronous communication is often called tightly coupled because all executions depend on each other. In contrast, asynchronous communication is called loosely coupled because asynchronous operations are independent.
What Is Synchronous And Asynchronous With Example
Lets evaluate what’s the difference between synchronous and asynchronous programming. In this case, we’ll present a JavaScript synchronous vs asynchronous example.
For the sake of simplicity, let’s imagine we want to sum two numbers and then display the result in a webpage’s HTML element.
In a synchronous project, this is how we could do it:
function performOperation(num1, num2) {
let sum = num1 + num2;
return sum;
}
value = performOperation(1, 2);
document.getElementById("example").innerHTML = value;
Fairly straightforward. We perform the operation, have the value returned to us, and then do what we want with it (in this case, display it).
Asynchronicity in JavaScript can be accomplished through the use of callback functions. Consider our example now done asynchronously:
function changeValueDisplay(value){
document.getElementById("example").innerHTML = value;
}
function performOperation(num1, num2, callback) {
let sum = num1 + num2;
callback(sum);
}
performOperation(1, 2, changeValueDisplay);
Notice the difference? We’re not explicitly displaying the value after performOperation. This is because performOperation is now responsible for calling a callback (in this case, changeValueDisplay) after the operation is complete to display the calculated value. You can easily imagine a more complex operation in place of our sum would severely slow down the page loading were this a synchronous operation. Now the programmer is no longer concerned with how long the operation takes, but the callback guarantees that after it’s done, however long it takes, the display will be changed.
Is Asynchronous Better Than Synchronous?
The answer depends. When considering async vs sync processing, you have to take into account the type of project you’re developing. Some types of processing are naturally more suited to certain types of projects. Let’s look at some advantages and disadvantages of each and see which is better in what situations.
Synchronous vs Asynchronous: Advantages and Disadvantages
Comparison | Synchronous Programming | Asynchronous Programming |
---|---|---|
Architecture | Single-thread, blocking | Multi-thread, non-blocking |
Execution | Sequential, one task at a time | Concurrent, multiple tasks can run simultaneously |
Advantages | Simplicity, easier to understand and implement | Faster, more responsive, scalable, better user experience |
Disadvantages | Can be slow, unresponsive with heavy computations | Increased code complexity, potential for operation overload |
Ideal Use Cases | Tasks that are computationally heavy or dependent and cannot run in parallel (e.g., web pages, video rendering) | Independent tasks, or tasks that take a long time and can run in the background (e.g., database manipulation, complex dashboards) |
Coding Complexity | Easier, straightforward | More complex, requires careful tracking and managing of processes |
Resource Intensity | Can be resource-intensive, slower | Increases throughput, allows more operations simultaneously |
Flexibility | Strict sequence of tasks | Adaptable, tasks can be executed without fixed sequence |
Synchronous has one main advantage:
- A simple, default method of programming: Synchronous programming is by far the simplest method and thus the default for many programs and also learning material. You need very little setup to do a project with this methodology.
But has one disadvantage as well:
- Can be slow: A synchronous project can become slow and unresponsive if it depends on computational-heavy algorithms, or a lot of operations in succession.
While Asynchronous has some key advantages:
- Faster and more responsive: A program that has operations running in different threads can become faster and present information to the user as it is processed, never letting the project become unresponsive and thus providing a better user experience.
- Better error handling: If an operation running in a different thread fails for some reason, you can handle that error or anomaly without shutting down the entire project.
- Higher scalability: By offloading operations to different threads, you can easily add new features or scale up your processing power to handle more requests.
But also comes with some disadvantages:
- Code complexity: This is the biggest disadvantage asynchronous programming brings. Your code becomes vastly more complex and harder to debug. Also, depending on the language you’re using, asynchronous programming operations can either be relatively easy or a nightmare.
- Operation overload: Take care not to overdo it with threads. Your processing can easily get swamped and then your project’s asynchronicity becomes a hindrance, rather than a boon.
Synchronous vs Asynchronous: Use Cases
You’ll want synchronous programming when your project focuses on a single task (especially if it’s computationally heavy), or tasks that are highly dependent and cannot run in parallel:
- Web Pages: By loading a web page synchronously, you make it easier for Search Engine Optimization (SEO) engines to find and categorize your page’s content.
- Video Rendering: This is a task that takes a lot from your CPU (if not most of it), and thus running other tasks in parallel would oversaturate it.
You’ll want asynchronous programming when you have a lot of tasks that do not depend on each other, or tasks that take a long time and can be run in the background:
- Database Manipulation: Databases, especially large ones, are notorious for the long times queries take when trying to retrieve information from them. By using a different thread for that job, you can let the rest of your application function while you wait for the results.
- Dashboards: If you have a complex dashboard with a lot of information to present, you can use different threads to keep each part updated in real-time or as the system can handle.
As a final note, if your project is relatively simple, using asynchronous programming is highly overkill and is generally not advised. Asynchronicity’s speed benefits would be countered by the inherent increase in complexity for your project.
Choosing the Right Approach between Synchronous and Asynchronous Programming
If you’re new to programming or handling a project that involves software development, deciding between synchronous and asynchronous programming can be daunting. Consider synchronous programming if your project requires tasks to be completed in a specific order, such as a web page where each element loads sequentially for better SEO. It’s more straightforward, making it a good starting point for beginners.
On the other hand, if your project involves tasks that can run independently without affecting each other, like updating different parts of a complex dashboard, asynchronous programming might be more suitable. It allows for more flexibility and responsiveness but is more complex in terms of code management.
Always weigh the complexity of your project against the benefits each method offers. For simple, sequential tasks, stick with synchronous programming. For complex, independent tasks where speed and responsiveness are crucial, consider asynchronous programming. Remember, the key is to match the programming approach to your project’s specific needs.
Conclusion
Asynchronous programming can become a great boon to a complex project, as well as a headache if it’s not handled properly. We hope this article was enlightening when it comes to the differences between synchronous and asynchronous programming, and where best to apply each of them. If you’re looking for developers for your project, be it synchronous or asynchronous, DistantJob has a large number of expert developers for hire. We have a strict and rigorous process that helps our clients find the best candidates that fit any company’s needs and culture fit, so don’t hesitate to contact us!